함수
특정한 일을 수행하는 코드 블록
평균, 순위, 합격자 수, 둘 중 큰 값, 90점 이상 점수 개수와 같이 특정 값 한 개를 많이 구하는 데 많이 사용한다.
종류가 2개가 있는데 반환값이 있는 함수와 반환값이 없는 함수로 나뉜다.
#include <math.h>가 필요한 수학 함수
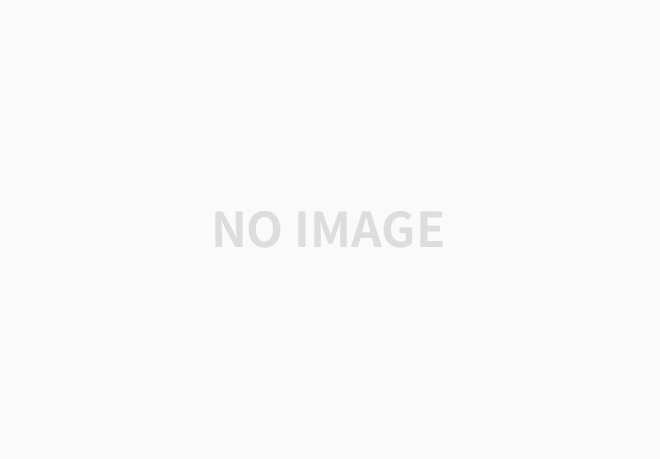
#include <stdlib.h>가 필요한 범용 함수
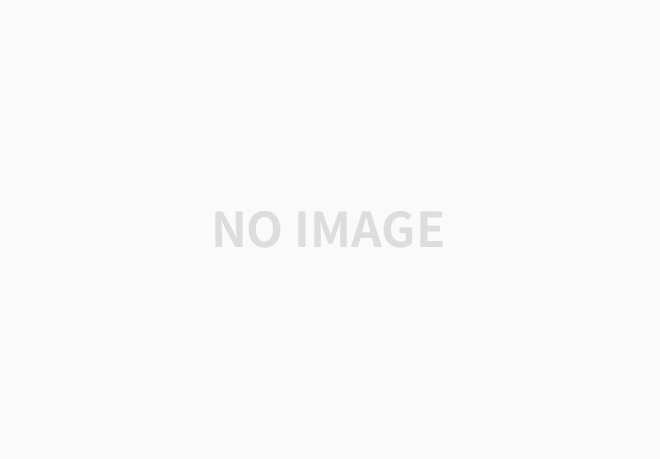
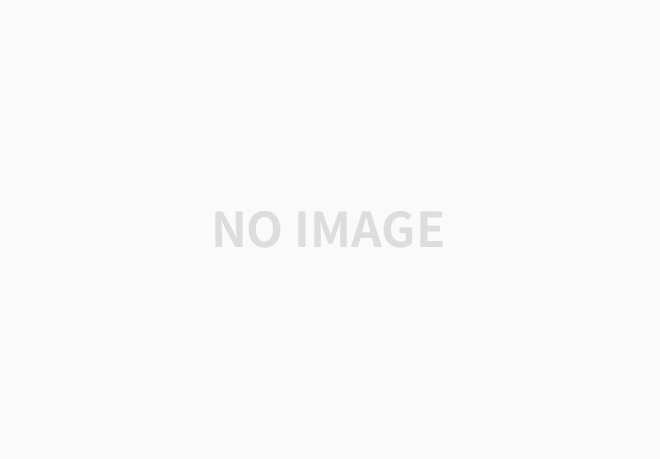
함수의 원형 선언
함수의 원형 선언이 필요한 경우의 예시가 있고 그렇지 않은 예시가 있다.
보통 함수의 원형 선언이 필요한 경우를 권장하는 편이다.
줄 출력하기 예시를 통해 알아보자.
함수의 원형 선언이 필요한 경우
#include <stdio.h>
#pragma warning(disable: 4996)
#pragma warning(disable: 6031)
void f_line();
int main()
{
printf("줄 출력하기\n");
f_line();
f_line();
return 0;
}
void f_line()
{
int i;
for (i = 0; i < 20; i++)
{
printf("=");
}
printf("\n");
return;
}
함수의 원형 선언이 필요하지 않은 경우
프로그램의 전체의 흐름은 main() 함수에 있으므로 main() 함수 정의를 맨 위에 두는 것이 바람직하며, 함수 원형을 main() 함수 위에 모두 모으면 이 프로그램에 포함된 함수로 어떤 것들이 있는지 쉽게 파악할 수 있으며, 함수를 호출할 때 해당 함수의 원형을 빠르게 찾아 참고하여 함수를 정확하게 호출할 수 있기 때문이다.
#include <stdio.h>
#pragma warning(disable: 4996)
#pragma warning(disable: 6031)
void f_line()
{
int i;
for (i = 0; i < 20; i++)
{
printf("=");
}
printf("\n");
return;
}
int main()
{
printf("줄 출력하기\n");
f_line();
f_line();
return 0;
}
주사위 눈의 수 맞히기, 숫자 암기, 구구단 게임
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#include <Windows.h>
#pragma warning(disable: 4996)
#pragma warning(disable: 6031)
void game_die();
void game_memory();
void game_99();
int main()
{
int game;
printf("어떤 게임을 할까요(1. 주사위 2. 숫자 암기 3. 구구단)? ");
scanf("%d", &game);
srand(time(NULL));
switch (game)
{
case 1: game_die(); break;
case 2: game_memory(); break;
case 3: game_99(); break;
default: printf("잘못 입력했습니다.");
}
return 0;
}
void game_die()
{
int num, die_spot;
die_spot = rand() % 6 + 1;
printf("\n주사위를 던졌습니다. 얼마일까요(1 ~ 6)?");
scanf("%d", &num);
die_spot == num ? printf("\n%d인지 어떻게 알았죠? 찍기 왕이군요!", die_spot) :
printf("\n%d인데 아쉽군요!\n", die_spot);
}
void game_memory()
{
int n1, n2, n3, r1, r2, r3;
n1 = rand() % 900 + 100;
n2 = rand() % 900 + 100;
n3 = rand() % 900 + 100;
printf("\n화면에 나타난 값 3개 암기하기!!\n");
printf("아무 키나 누르면 시작해요. ");
getch(); system("cls");
printf("\n%4d %4d %4d \n", n1, n2, n3);
Sleep(2000); system("cls");
printf("무슨 값(차례대로 빈칸으로 구분)? ");
scanf("%d %d %d", &r1, &r2, &r3);
(n1 == r1) && (n2 == r2) && (n3 == r3) ?
printf("\n암기력 짱! \n") :
printf("\n아쉽게도 틀렸네요~ \n");
return;
}
void game_99()
{
int n1, n2, reply;
printf("구구단 게임입니다.\n");
n1 = rand() % 8 + 2;
n2 = rand() % 8 + 2;
printf("%d * %d = ?", n1, n2);
scanf("%d", &reply);
reply != n1 * n2 ?
printf("%d인데 틀렸습니다! ㅋㅋ \n", n1 * n2) :
printf("맞았습니다. ^^ \n");
return;
}
'프로그래밍 > C,C++' 카테고리의 다른 글
문자열 처리 (0) | 2024.05.28 |
---|---|
인수 전달하는 함수 (0) | 2024.05.28 |
자료 배열 2 (9장) (0) | 2024.05.27 |
자료 배열 1 (8장) (0) | 2024.04.29 |
C,C++ - 제어문 (while, do~while문) (0) | 2024.04.08 |