1. 파일 열기
open("파일명","r",encoding = 'utf-8')
open("파일명","w")
open("sample.txt", "rt") # 파일없으면 error남.
open("sample.txt", "wt") # 파일없어도 error X.
# 뒤에 t는 text로 읽고 쓰겠다는 뜻
주피터랩 코드설명: shift + tab
파일 열기 모드 (open("파일명", 모드, encoding, .....) 여기서 mode에 들어가는 것들을 말함.)
- 생략 : r과 동일
- r : 읽기 모드, 기본값
- w: 쓰기 모드, 기존에 파일이 있으면 덮어씀.
- r+ : 읽기/ 쓰기 겸용 모드
- a : 쓰기 모드. 기존에 파일이 있으면 이어서 씀. append의 약자
- t : 텍스트 모드. 텍스트 파일을 처리. 기본값
- b: 바이너리 모드. 바이너리 파일(=이진 파일)을 처리
2. 파일처리
- 파일에 데이터를 쓰거나 파일로부터 데이터를 읽어옴.
3. 파일 닫기
변수명.close()
+ 컴퓨터가 파일을 가져오는 과정
CPU > memory > disk
disk > memory > CPU
자료가 나타나고 저장하는과정
파일을 열었으면 닫아줘야함.
다 읽으면 메모리 낭비가 심할 수 있음.
텍스트 파일 입력 (읽기)
read() : 파일 전체를 읽어 문자열로 return 해줌.
file_example = open("data.txt", 'rt')
data_string = file_example.read()
print(data_string)
file_example.close()
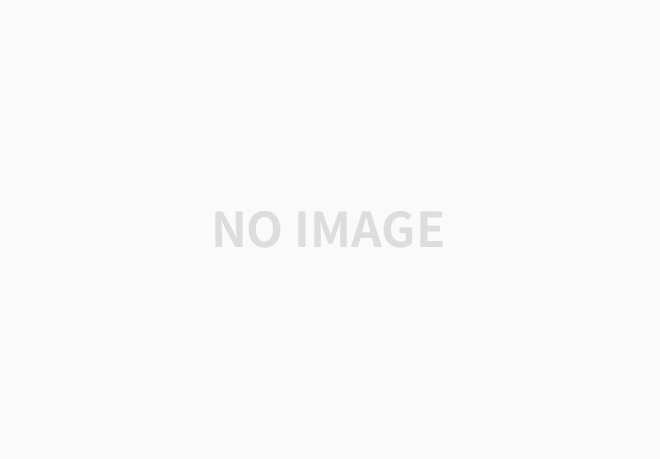
readline() : 한 줄을 읽어 문자열로 return
file_example = open("data.txt", 'rt')
line_string = file_example.readline()
print(line_string)
file_example.close()
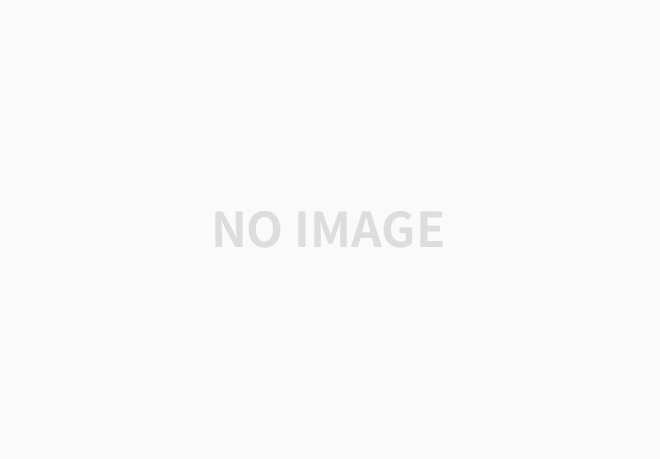
readlines() : 파일 전체를 읽어 줄 문자열이 원소인 list 형태로 return
file_example = open("data.txt", "rt")
line_list = file_example.readlines()
print(line_list)
file_example.close()
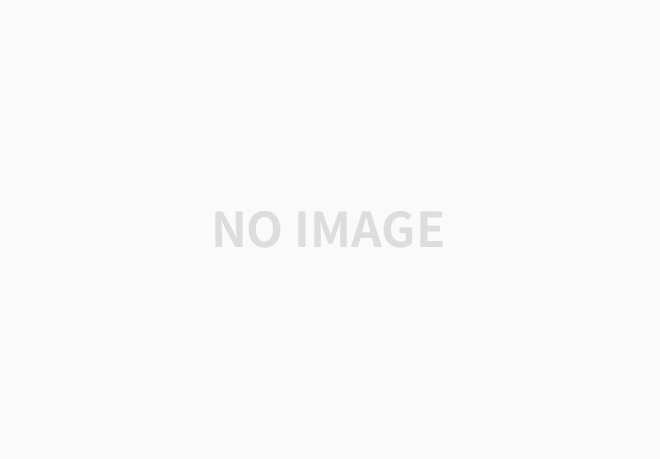
여기서 보면 각각의 원소 뒤에 \n이 붙어있는 것을 확인할 수 있다.
그래서 각각의 원소가 한 줄 띄우고 출력될 것이라는 것을 예상할 수 있는데
실행해보면 다음과 같이 나온다.
# 각각의 원소의 결과값을 알기 위해 실행하는 코드
file_example = open("data.txt", "rt")
line_list = file_example.readlines()
for line in line_list:
print(line)
file_example.close()
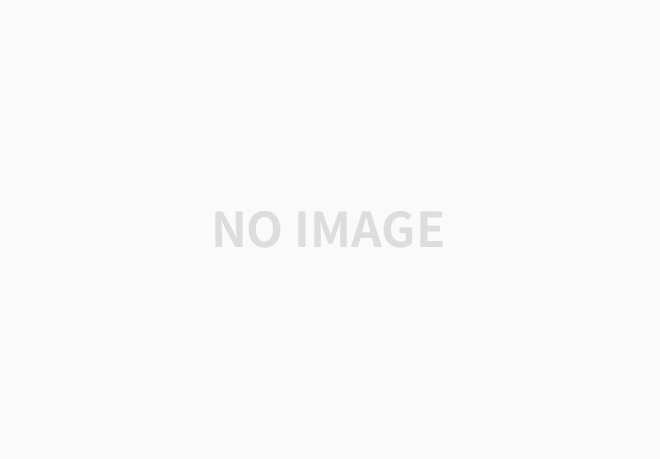
이렇게 출력되는데 이것은 print가 default값으로 \n을 가지기 때문이다.
이걸 해결해주기 위해 다음과 같은 코드를 짤 수 있다.
# 각각의 원소의 결과값을 알기 위해 실행하는 코드
file_example = open("data.txt", "rt")
line_list = file_example.readlines()
for line in line_list:
line = line.strip("\n")
print(line)
file_example.close()
'프로그래밍 > Python' 카테고리의 다른 글
Python - File Encoding, 자동으로 파일 객체 닫기 (2) | 2023.10.21 |
---|---|
Python - file open 시 오류 처리 (0) | 2023.10.21 |
Python - 피보나치 수열 (0) | 2023.10.14 |
Python - 재귀함수 (0) | 2023.10.14 |
Python - 모듈, 예외처리 (1) | 2023.10.14 |